Developer Getting Started Guide: Using the Compass Context API
This tutorial helps you harness the power of the Compass Context API to analyze claims and provide detailed contextual insights with step-by-step Python examples for quick integration.
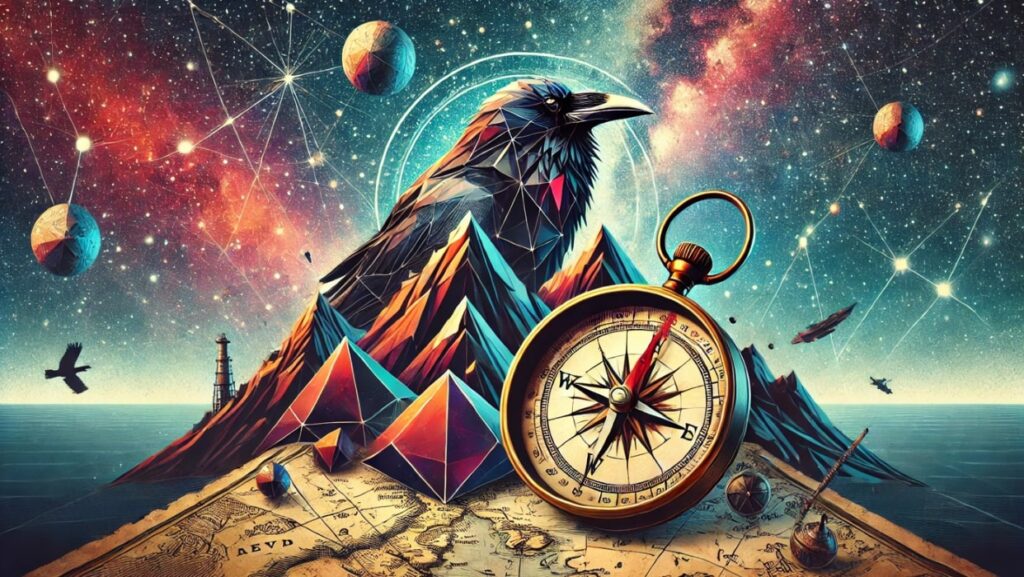
The Compass Context API enables developers to analyze online claims and retrieve detailed contextual information, empowering users to make informed decisions. This tutorial introduces the API with step-by-step examples for creating and retrieving context checks in Python.
What is Compass Context?
Compass Context is an AI-powered tool to analyze claims in articles, social media posts, and other content types. It provides users with:
- Contextual Analysis: Detailed insights into the validity of claims.
- Multiple Input Types: Supports text, web links, social media content, images, and videos.
- Source Citations: References for every analysis, ensuring transparency.
LEARN: What Is Narrative Intelligence?
Getting Started: Prerequisites
Getting started with the Compass Context API is simple. All you need is a Compass username and password, which you can obtain by creating an account or logging in at https://compass.blackbird.ai/.
Once you have your login credentials, you can use them to generate an authentication token required to access the API programmatically. No additional API keys or setup is needed. Because this API uses the same account as the Compass web app, your rate limit is shared across both platforms, and you can view your results in either the web app or the API.
The generated token expires after one hour. For long-lived access tokens or users requiring higher rate limits, please contact our enterprise team for more information.
The code examples in this tutorial are also available as a Colab notebook, and additional API documentation is available at https://docs.blackbird.ai/.
LEARN MORE: The World Economic Forum recently ranked narrative attacks created by misinformation and disinformation as the #1 global risk for the second consecutive year. Harmful narratives also fan the flames of the top five risks, including extreme weather, state-based armed conflict, societal polarization, and cyber espionage. Check out the report here.
API Workflow
The Compass Context API follows a straightforward, four-step workflow:
- Generate an Authentication Token: Authenticate using your username and password to obtain a short-lived (one-hour) access token.
- Create a Context Check: Submit a claim for analysis using the /contextChecks endpoint.
- Poll for Processing Status: Monitor the status of your context check with the /contextChecks/{id} endpoint.
- Retrieve Results: Once processing is complete, retrieve the full analysis, including a summary, source citations, and risk classification.
Generating an Authentication Token
To interact with the API, you must first generate an authentication token using your Compass username and password.
import requests
# Set your Compass credentials and token URL
context_token_URL = "https://api.blackbird.ai/compass/token"
context_username = "your_username"
context_password = "your_password"
def get_token():
"""Retrieve an authentication token."""
credentials = {
"username": context_username,
"password": context_password,
"grant_type": "password"
}
response = requests.post(context_token_URL, json=credentials)
return response.json().get("access_token")
# Example usage
auth_token = get_token()
print(f"Authentication Token: {auth_token}")
Output
On success, this function returns the access_token required for API requests. Include this token in the Authorization header of all subsequent API calls.
Creating a Context Check
Submit a claim to the /contextChecks endpoint for analysis. The request body must include:
- input: The text or claim to analyze.
Example Request
# Set up the API endpoint and authentication token
api_url = "https://api.blackbird.ai/compass/contextChecks"
auth_token = "your_access_token"
def create_context_check(claim):
"""Submit a claim for context analysis."""
headers = {
"Authorization": f"Bearer {auth_token}",
"Content-Type": "application/json",
}
payload = {
"input": claim
}
response = requests.post(api_url, headers=headers, json=payload)
return response.json().get("id")
# Example Usage
claim = "The Eiffel Tower is burning."
context_check_id = create_context_check(claim)
print(f"Context Check ID: {context_check_id}")
Polling for Processing Status
After creating a context check, use the /contextChecks/{id} endpoint to monitor its status. For example:
{
"id": "19068",
"created_at": "2025-01-16T14:50:15.107Z",
"updated_at": "2025-01-16T14:50:15.107Z",
"status": "processing",
"input": "The Eiffel Tower is burning."
}
- status: Indicates whether the context check is still processing or completed as success.
- id: The unique identifier for the context check.
- input: The claim submitted for analysis.
- created_at / updated_at: Timestamps for tracking progress.
Poll periodically until the status changes to success.
Retrieving Results
Once processing is complete, retrieve the full results of the context check. The response includes:
- summary: A concise explanation of the claim’s validity.
- sources: References supporting the analysis.
- label: A risk classification (Safe, Caution, or Warning).
Example Request
def get_context_check(context_check_id):
"""Retrieve the results of a context check."""
url = f"https://api.blackbird.ai/compass/contextChecks/{context_check_id}"
headers = {"Authorization": f"Bearer {auth_token}"}
response = requests.get(url, headers=headers)
return response.json()
# Example Usage
result = get_context_check(context_check_id)
print("Context Analysis Result:", result)
Example Output
{
"id": "19067",
"status": "success",
"input": "The Eiffel Tower is burning.",
"context": {
"summary": "The Eiffel Tower is not burning; reports of a fire are false and have been debunked.",
"sources": [
{"url": "https://www.newsweek.com/eiffel-tower-fire-today-latest-update-2005595"},
{"url": "https://www.euronews.com/travel/2024/12/24/tourists-evacuated-from-eiffel-tower-after-reports-of-elevator-fire"},
{"url": "https://www.reuters.com/fact-check/fake-photo-shows-eiffel-tower-ablaze-2024-01-18/"}
],
"label": "Warning"
}
}
The Way Forward – Four Takeaways For Developers
Start using the Compass Context API to integrate claim analysis into your applications. Explore additional endpoints for further capabilities, such as image and video analysis.
Integrate Context Analysis into Your Applications
Leverage the power of the Compass Context API to analyze claims and deliver real-time contextual insights. Start by creating an account or logging in at Compass by Blackbird.AI and access API documentation to begin integration.
Explore Advanced Capabilities Beyond Claim Analysis
Expand your application’s verification capabilities with additional endpoints for analyzing images, videos, and social media content. Visit the Compass Context API Documentation to explore these powerful tools.
Enhance Trust and Transparency with Source Citations
Build credibility with source-backed claim analysis by using the API’s citation features. Learn how Blackbird.AI’s solutions support transparency and informed decision-making at Blackbird.AI Solutions.
Stay Ahead with Blackbird.AI’s Expertise
Access thought leadership, case studies, and industry reports to deepen your understanding of how AI-driven tools like Compass Context transform digital trust. Explore resources at the Blackbird.AI RAV3N Blog and stay updated on new developments.
For more details, please read the API documentation.
- To receive a complimentary copy of The Forrester External Threat Intelligence Landscape 2025 Report, visit here.
- To learn more about how Blackbird.AI can help you in these situations, book a demo.
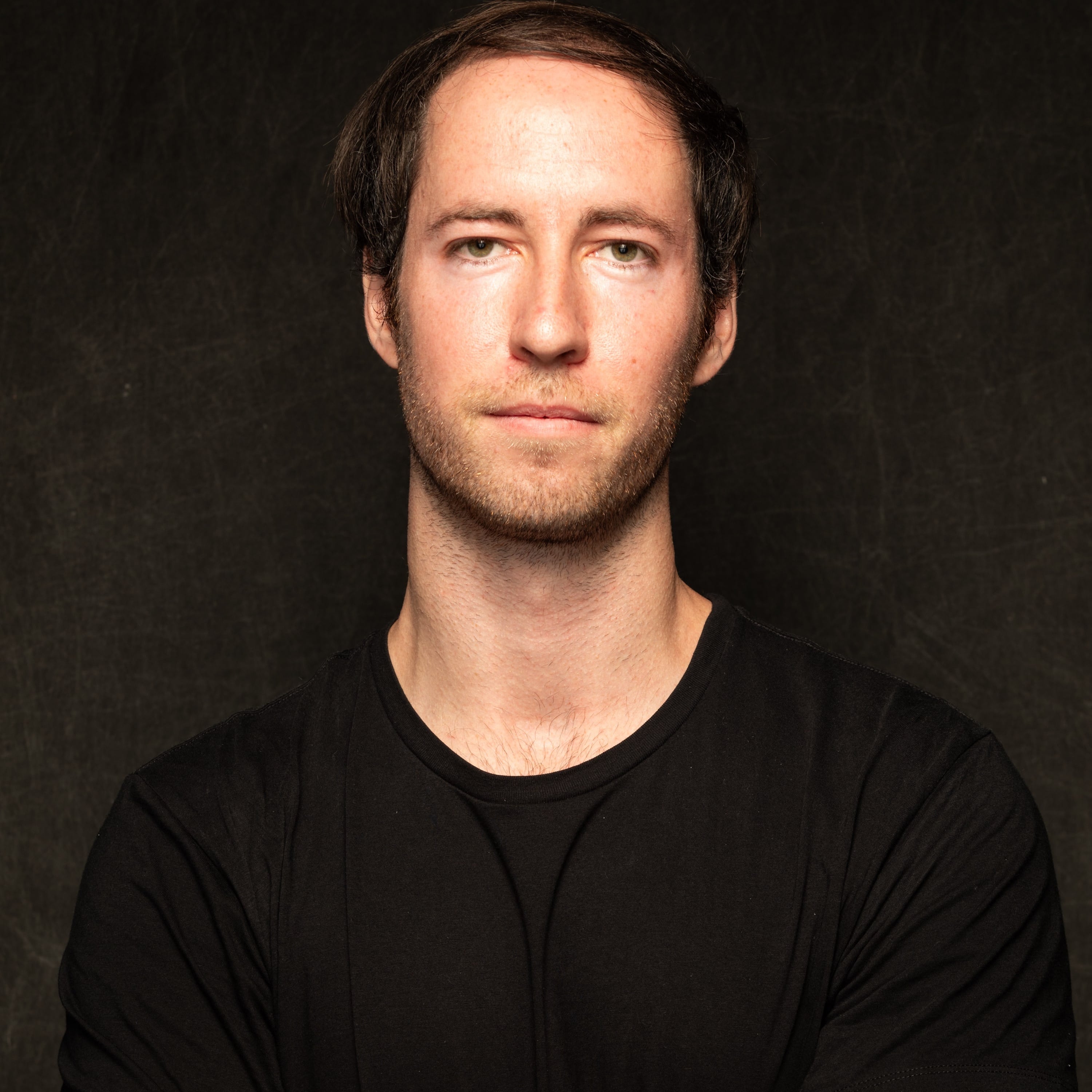
Vanya Cohen • Principal AI Scientist
Vanya Cohen is Blackbird.AI’s Principal AI Scientist. His research and work are focused on applying generative AI to mitigate narrative attacks caused by misinformation and disinformation. Vanya leads the development of Compass by Blackbird.AI, a multimodal LLM-powered agent that analyzes claims made in social media posts, news articles, images, and videos.
Vanya Cohen is Blackbird.AI’s Principal AI Scientist. His research and work are focused on applying generative AI to mitigate narrative attacks caused by misinformation and disinformation. Vanya leads the development of Compass by Blackbird.AI, a multimodal LLM-powered agent that analyzes claims made in social media posts, news articles, images, and videos.
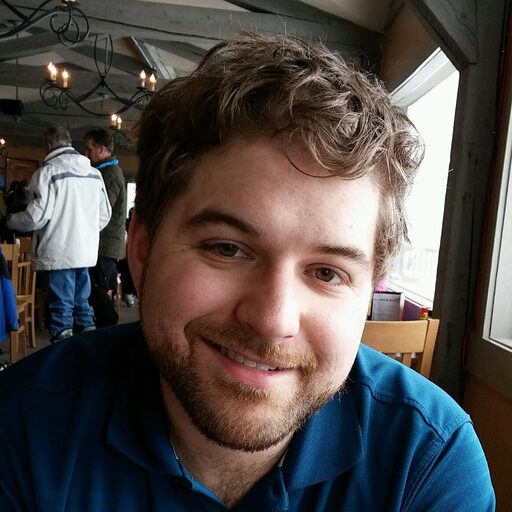
Zachary Marois • Software Engineer
Zach is a ninja engineer (ninjineer?) who covertly injects into any operation, gets the job done well, and leaves with no mess behind. His hobbies include skiing, puzzles, and magnets.
Zach is a ninja engineer (ninjineer?) who covertly injects into any operation, gets the job done well, and leaves with no mess behind. His hobbies include skiing, puzzles, and magnets.
Need help protecting your organization?
Book a demo today to learn more about Blackbird.AI.